For anyone getting this exception while reading data from the stream, this may help. I was getting this exception when reading the HttpResponseMessage in a loop like this:
using (var remoteStream = await response.Content.ReadAsStreamAsync())
using (var content = File.Create(DownloadPath))
{
var buffer = new byte[1024];
int read;
while ((read = await remoteStream.ReadAsync(buffer, 0, buffer.Length)) != 0)
{
await content.WriteAsync(buffer, 0, read);
await content.FlushAsync();
}
}
After some time I found out the culprit was the buffer size, which was too small and didn’t play well with my weak Azure instance. What helped was to change the code to:
using (Stream remoteStream = await response.Content.ReadAsStreamAsync())
using (FileStream content = File.Create(DownloadPath))
{
await remoteStream.CopyToAsync(content);
}
CopyTo() method has a default buffer size of 81920. The bigger buffer sped up the process and the errors stopped immediately, most likely because the overall download speeds increased. But why would download speed matter in preventing this error?
It is possible that you get disconnected from the server because the download speeds drop below minimum threshold the server is configured to allow. For example, in case the application you are downloading the file from is hosted on IIS, it can be a problem with http.sys configuration:
«Http.sys is the http protocol stack that IIS uses to perform http communication with clients. It has a timer called MinBytesPerSecond that is responsible for killing a connection if its transfer rate drops below some kb/sec threshold. By default, that threshold is set to 240 kb/sec.»
The issue is described in this old blogpost from TFS development team and concerns IIS specifically, but may point you in a right direction. It also mentions an old bug related to this http.sys attribute: link
In case you are using Azure app services and increasing the buffer size does not eliminate the problem, try to scale up your machine as well. You will be allocated more resources including connection bandwidth.
Have you recently been facing the “An existing connection was forcibly closed by the remote host” error on your system? This problem usually happens when you try connecting with any remote host, and the process fails.
After going through the various complaints and grievances reported by the users, we looked into the issue and carved out the best solutions.
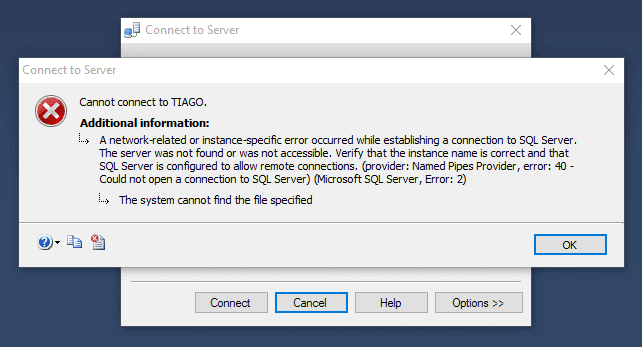
Be patient and read the article.
What Are the Reasons Behind the “An Existing Connection Was Forcibly Closed by the Remote Host” Error?
The lack of a physical presence characterizes the remote computer. This means that the only way to access this computer is through a computer network. The computer that hosts the network for the particular remote computer is called the Remote Host.
The user that operates the remote computer through the network is called the Remote Client. This is a great feature and revolutionizes computing processing.
However, the host and client connection has recently been facing errors.
But Before We Begin, Let Us Also Take You Through the Causes That Are the Potential Problem-Makers so That You Do the Fixing Better:
- Disabled cryptography – If you have not noticed that the feature of Cryptography is disabled on the machine you are using, it will hinder the machine from using TLS 1.2, and it will get the application back to TLS 1.0 usage. This auto depreciation triggers the error.
- The usage of TLS 1.0 / 1.1 – If the application uses TLS 1.0 or TLS 1.1 for operation and storage, there is a high chance that the depreciated TLSs are causing the error. The right type of TLS to be used is TLS 1.2, while selecting the right protocol to be used by the application.
- Faulty socket implementation – There are some cases where a specific socket implementation becomes the reason behind the lost connection error. The associated .NET application that causes the implementations might have got some bugs and become the troublemaker.
- Lost code – Some users working with the Entity Framework also faced the error. This was caused by a specific line of code that was lost, causing trouble in the operation of the application.
- An older .NET Framework – In some cases, the “An existing connection was forcibly closed by the remote host” error was being caused. The reason was the .NET framework being disabled. Some system tasks need the latest framework version installed on the PC to run properly. If the condition is not fulfilled, then they cease to function.
Five Best Methods to Fix the Connection Error
Pick any of these solutions per the issue causing the error.
Besides, here, you will figure out what is the ETD Control Center.
Method 1: Enable the Cryptography
One of the prime reasons you have seen the error message saying, “An existing connection was forcibly closed by the remote host,” is that TLS 1.2 is prohibited from running on the machine.
This hindered the Cryptography from being operational thereby and caused the problem. A logical solution is to enable Cryptography, which will potentially solve the issue.
Below is the series of steps that you need to follow to enable the Cryptographic operation:
1. Press the keys Windows + R together to launch the prompt of the Run Dialogue box.
2. Enter the command regedit. Now hit the button to enter.
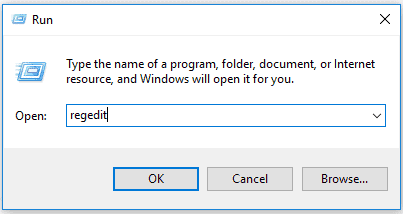
3. Navigate down and then find the address:
KEY_LOCAL_MACHINESOFTWAREMicrosoft.NETFrameworkv4.0.3031
4. Look inside the right pane, and if you don’t find any value like “SchUseStrongCrypto,” then you will have to find this address:
HKEY_LOCAL_MACHINESOFTWAREWow6432NodeMicrosoft.NETFrameworkv4.0.30319
5. Again, look through the right window pane and hit right-click over the option of “SchUseStrongCrypto.” Then enter the number 1 as the value of the data being entered.
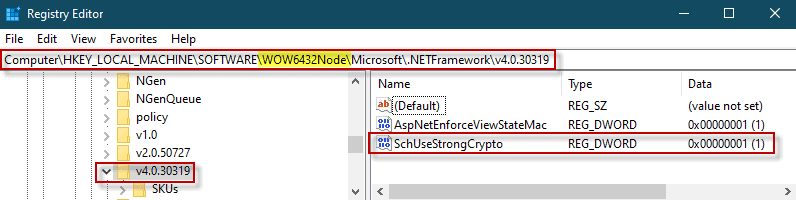
6. Lastly, click on the option OK to save the changes you made successfully.
Now go back to the operation you were running previously and see if the error is gone.
Method 2: Force Launching the Usage of TLS 1.2
It might not have come to your notice, but the application you have been trying to run might have experienced certain changes in its configuration.
Sometimes the user settings of any application are set at TLS 1.0 or TLS 1.1, while the correct option should be TLS 1.2.
This change can become the reason for the error “An existing connection was forcibly closed by the remote host.”
This method will deal with the error by changing such system configurations and setting it to TLS 1.2. Follow the steps given below:
1. Navigate and find the root folder of the particular website. Now hit a right-click over the file with the name global.asax.
2. Select the option of View Code from the given list.
3. Find the option of the method named ‘Application_Start‘ that must be in the next window pane. The line of code that we give you down below needs to be added in the particular method:
if (ServicePointManager .SecurityProtocol.HasFlag (SecurityProtocoType.Tls12) == false)
{
ServicePointManager.SecurityProtocol = ServicePointManager.SecurityProtocol | SecurityProtocolType.Tls12;
}
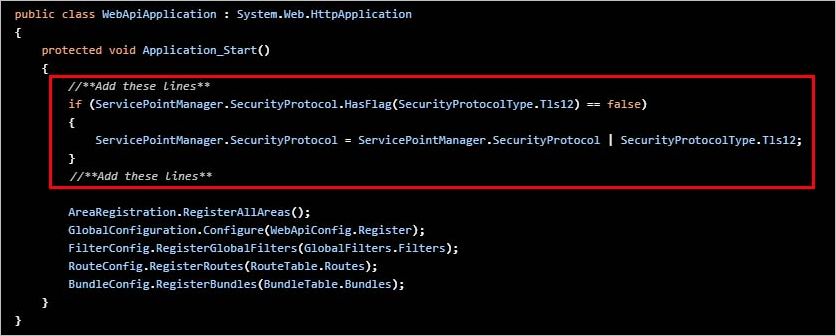
4. Save these changes that you made.
Go back to running the previous operation and see if the method has removed the error.
Method 3: Change the Socket Implementation
A faulty socket implementation is another probable reason why the error “An existing connection was forcibly closed by the remote host” has been creating trouble with the program you wanted to operate.
Sometimes, any glitch or bug that might have affected the socket implementation related to the program happens.
This hinders the program’s concerning elements from running smoothly, and the error, as mentioned earlier, is the result. In this method, we sought to configure and set up a new implementation, which will be different from the previous one.
Follow the steps we give below:
1. Before starting the process, check properly and ensure that the class StateObject is available to you. The code “public byte[] buffer = new byte[1024], public Socket socket;” should be there too.
2. Make a call over the function named “Receive (Socket s)” and then call in the mentioned code “void ReceiveCallback(IAsyncResult ar)“
SocketError errorCode;
Int nBytesRec = socket.EndReceive (ar, out errorCode);
If (errorCode != SocketError.Success)
{
nBytesRec = 0;
}
Once you have successfully implemented this code, go back, and see if the error remains. It would probably be solved by now.
If it is not, move ahead with the next methods that we give you for dealing with the error “An existing connection was forcibly closed by the remote host.”
Method 4: Add the Command Lines (for the Users of Entity Framework)
If you have been a user of the Entity Framework, then there might be a possibility that a particular line of code must have been left out.
We aim to deal with this issue in this method, where we will add the missing line of code to fix the final host error issue.
Follow the steps below to do so:
1. Open the file named ‘.edmx.’ on your system. You will find a file named ‘.context.tt‘ below the previous file. Open it.
2. Now open the file named ‘.context.cs‘. In the associate constructor, you need to enter the line of code that we give you below:
Public DBEntities ()
: base(“name=DBEntities”)
{
This.Configuration.ProxyCreationEnabled = false; // ADD THIS LINE !
}
Once the command runs successfully, go back to the application you were trying to open and see if it has gone error-free.
Method 5: Update the .NET Framework
Many elements need to operate nicely for any application to run smoothly. The .NET framework is one such component, and you need to have the latest version of this framework installed on your PC to ensure the proper functioning of the related applications.
Therefore, this method will deal with installing the updated version of the .NET framework from the right website.
Follow the steps to do so:
1. Open Microsoft’s official website to download the setup of the concerned framework. Finish the download and begin to install the setup properly.
2. Find the .exe file in the setup and open it to execute it. This will begin the process of installing the framework program.

3. Keep following the instructions as they appear on the screen for installing the application properly on the PC that you are using.
Now go back to run the program that you were previously trying to. See if this method successfully removes the error “An existing connection was forcibly closed by the remote host.”
Conclusion
The error “An existing connection was forcibly closed by the remote host” hampers the connection between the server and the client. We hope that the solutions helped rectify the error and eliminate it.
A remote computer is the one which has no physical presence; it can be accessed only through some sort of a computer network. The Remote Host is the computer hosting the network which hosts the remote computer and the remote client is the user of the remote client on the network. This feature has revolutionized a lot of processes and has a great scope in the future as well.
However, quite recently, a lot of reports have been coming in of an “an existing connection was forcibly closed by the remote host” error while trying to connect to the remote host. This error is triggered with a socket connection between a client and a server. In this article, we will provide some viable solutions to completely rectify this error and also inform you of the reasons that trigger this error.
What Causes the ‘An existing connection was forcibly closed by the remote host’ Error in Windows?
After receiving numerous reports from multiple users, we decided to investigate the issue and devised a set of solutions to fix it. Also, we looked into the reasons due to which it is triggered and listed them below.
- TLS 1.1/1.0 Usage: If the application is running on TLS 1.1 or TLS 1.0, it might trigger this error due to them being depreciated. TLS 1.2 is the way to go when selecting the protocol which the application uses.
- Cryptography Disabled: If Cryptography has been disabled for your machine it will prevent the usage of TLS 1.2 and will fall back on TLS 1.0 which might trigger the error.
- Socket Implementation: In some cases, a particular type of socket implementation triggers the error. There is a bug with some implementations by “.NET” application and it might cause this error.
- Missing Code: For some people who were using the Entity Framework, it was observed that a certain line of code was missing due to which the error was being triggered.
- Outdated “.NET” Framework: In certain cases, if the “.NET” Framework has been disabled, this error might be triggered. Certain tasks require the “.NET” framework to be updated to the latest version in order for them to work properly.
Now that you have a basic understanding of the nature of the problem, we will move on towards the solutions. Make sure to implement these in the specific order in which they are presented to avoid conflicts.
Solution 1: Enabling Cryptography
If Cryptography has been disabled for your machine the usage of TLS 1.2 is prohibited. Therefore, in this step, we will be enabling Cryptography. For that:
- Press “Windows” + “R” to open the Run prompt.
- Type in “regedit” and press “Enter“.
Typing in “Regedit” and pressing “Enter” - Navigate to the following address
HKEY_LOCAL_MACHINESOFTWAREMicrosoft.NETFrameworkv4.0.3031
Navigate to this address if there is no “SchUseStrongCrypto” value in the right pane.
HKEY_LOCAL_MACHINESOFTWAREWow6432NodeMicrosoft.NETFrameworkv4.0.30319
- In the right pane, double click on the “SchUseStrongCrypto” option and enter “1” as Value data.
Double clicking on the “SchUseStrongCrypto” value in the right pane - Click on “OK” to save your changes and check to see if the issue persists.
Solution 2: Forcing TLS 1.2 Usage
If the application has been configured to use TLS 1.1 or TLS 1.0 instead of the TLS 1.2, it might trigger this error. Therefore, in this step, we will be configuring our computer to use TLS 1.2. For that:
- Navigate to the root of the site and right-click on the “global.asax” file.
- Select “View Code” from the list.
- There should be an “Application_Start” method, add the following line of code to that method
if (ServicePointManager.SecurityProtocol.HasFlag(SecurityProtocolType.Tls12) == false) { ServicePointManager.SecurityProtocol = ServicePointManager.SecurityProtocol | SecurityProtocolType.Tls12; }
Adding the lines to the code - Save your changes and check to see if the issue persists.
Solution 3: Changing Socket Implementation
If a certain socket implementation has a bug or glitch in it, it might prevent certain elements of the application from functioning properly due to which this error might be triggered. Therefore, in this step, we will be configuring it to use a different implementation. For that:
- Make sure you have a “StateObject” class with “public byte[] buffer = new byte[1024], public Socket socket;“.
- Call the “Receive(Socket s)” function and call the following code in “void ReceiveCallback(IAsyncResult ar)”
SocketError errorCode; int nBytesRec = socket.EndReceive(ar, out errorCode); if (errorCode != SocketError.Success) { nBytesRec = 0; }
- Check to see if the issue persists after implementing this code.
Solution 4: Adding Command Lines (Only for Entity Framework)
If you are using the Entity Framework, it is possible that a certain line of code might be missing. Therefore, in this step, we will be adding that line of code in order to fix this issue. For that:
- Open your “.edmx” file and open the “.context.tt” file below it.
- Open the “.context.cs” file and add the following line of code to your constructor
public DBEntities() : base("name=DBEntities") { this.Configuration.ProxyCreationEnabled = false; // ADD THIS LINE ! }
- Check to see if the issue persists after adding this line of code.
Solution 5: Updating .NET Framework
The latest version of the “.NET” Framework is required in order for everything to function smoothly. Therefore, in this step, we will be downloading the latest version from the site and installing it. For that:
- Navigate to this link to download the setup.
- Execute the “.exe” file in order to start the installation process.
Running the executable downloaded from Microsoft - Follow the onscreen instructions to install the application on your computer.
- Check to see if the issue persists after completing the installation.
Kevin Arrows
Kevin Arrows is a highly experienced and knowledgeable technology specialist with over a decade of industry experience. He holds a Microsoft Certified Technology Specialist (MCTS) certification and has a deep passion for staying up-to-date on the latest tech developments. Kevin has written extensively on a wide range of tech-related topics, showcasing his expertise and knowledge in areas such as software development, cybersecurity, and cloud computing. His contributions to the tech field have been widely recognized and respected by his peers, and he is highly regarded for his ability to explain complex technical concepts in a clear and concise manner.
Having a problem, while accessing or logging into your Minecraft server? Sometimes you may have to suffer “An Existing Connection Was Forcibly Closed by the Remote Host” error.
The full code looks like this:
This message shows that there is a problem with your computer network to access the Minecraft server.
This situation looks quite awkward. But, It is hard to find this error and it is also hard to fix it. Because it may be due to some interim issue and fixes itself by some time.
What does mean of An Existing Connection Was Forcibly Closed by the Remote Host?
You see this error when you start your favorite game Minecraft to play. This error prevails when you try to log in and try to connect your Pc or laptop with the Minecraft server.
First, we will discuss the reasons for this error.
Reasons for the Error “An Existing Connection Was Forcibly Closed by the Remote Host”
This error can appear for many reasons. The most common reason for the error is a firewall blocking the connection, internet connection, and it may be due to router issues.
Here are some other reasons for this error.
- As a user of Minecraft is far away from where it’s server is hosted. Sometimes this causes their router to time out the connection and disconnects the user.
- Sometimes, NAT acceleration would be disabled for the router setting. This setting can be found in LAN > Switch Control.
- It may be caused by the Firewall blocking the connection, internet connection, or maybe router issues.
- Now we look at some possible fixes to this error. These solutions will helpful for you to solve the existing connection was forcibly closed by the remote host error.
Solutions to fix An Existing Connection Was Forcibly Closed by the Remote Host
Let us now discuss the solution to this error. It will help you to fix this situation.
Solution#1 Deactivate Windows Firewall
Windows Firewall is blocking the connection between your computer to the Minecraft server. The Firewall is blocking the connection, internet connection, and maybe router issues. Following is the procedure to fix An Existing Connection Was Forcibly Closed by the Remote Host Error
Step1: Click on the start menu and select the setting option
Step2: Open the Update and Security tab.
Step3: Choose the options windows security.
Step4: Find out and check out the option Firewall and network protection.
Step5: Click on your active network, that is active.
Step6: After that click on the toggle button to turn off the Window Firewall.
After that, open your Minecraft to see if the error is removed or not.
if the error exists then don’t worry there is another solution to fix the problem.
If the Java.io.IOException error disables then you have to do a small task to allow the Java SE Binary to allow the Firewall.
Here are the steps to follow.
Step1: Hold on the keys Windows+S.
Step2: Go to the control panel go to the system and security tab.
Step3: From there go to Windows Defender Firewall.
Step4: Click on Allow an app or feature through Windows Defender Firewall in the left pane.
Step5: From there go to the next page, click on the Change Settings button and find out Java Platform SE Binary.
Step6: Find out the Private box option. If you see more options of Java Platform mark then nominate all of them as private. In the last cluck OK to save the changes.
Then restart the computer and try to connect your PC with the server to see if the error is disappeared.
If the error contains then you need to jump on the next method.
Solution#2 Change the View Side of Server
The lower view side may help you to connect the server decently. To do this you have to follow these steps.
Step1: Stop if the server is running or working
Step2: Click on files.
Step3: choose Configuration File and then move on to the server setting.
Step4: Fund out the View Distance option.
Step5: Change the View Distance number from there. Set it ‘4’.
Step6: Save the setting.
Now restart your PC to check out if the error resolved. This option proves helpful for many users who suffer in this situation.
If the problem exists then move on to the next solution.
Solution#3 Change the DNS Address
Sometimes this problem is created because of the DNS Address. So you should check out this.
Here are the steps to find out the error.
Step1: Open the Control Panel and then move on to Network and Internet settings
Step2: Click on Change Adapter Setting.
Step3: Right-click on the network icon to open the Properties.
Step4: Move down and double click the Internet Protocol Version 4 option.
Step5: Choose the option Use the following DNS server addressed option.
Step6: Enter the input DNS public DNS address.
- Preferred DNS server:8.8.8.8
- Alter ate DNS server: 8.8.4.4
There Is another solution that will be helpful for you to fix the problem.
Solution#4 Reinstall Java
If you want to get rid of An Existing Connection Was Forcibly Closed by the Remote Host. Therefore, you can reinstall Java. We mention the steps below to reinstall Java.
Step1: Press and hold on the keys Windows+R to Open Run setting.
Step2: Type appwiz.CPL and click ok.
Step3: Select the Minecraft apps that you want to install.
Step4: Click on uninstall, and then click on ok.
Step5: After uninstalling, then reinstall the latest version of Minecraft.
If the error An Existing Connection Was Forcibly Closed by the Remote Host does not fix then you don’t worry. There Is another solution so move on it.
Solution#5 Reinstall Minecraft
There is a possibility that the error occurs due to the Minecraft game. Then you have to reinstall Minecraft.
Here are the steps to uninstall the Minecraft
Step1: Press Windows+R both keys together to run the setup.
Step2: Type appwiz. CPL and select the ok option.
Step3: Click on uninstall. Then select the ok option to confirm the action
Step4: Wait for installation then reinstall it.
Solution#6 Restart Your Network
If an existing connection was forcibly closed by the remote host in Minecraft exists then it might because of your network’s problem. So you have only one option left that Is to restart your network connection.
Follow below steps
- Check if the router or modem is on.
- Turn off the router or modem for a while.
- Plug out the internet cable from the device.
- Wait for a while, then plug in the cable and power on the router or modem.
- Make sure that all indicators on the router or modem are blinking.
- In the last connect your PC or another device to check if the problem is fix
We hope your issue is resolved.
Final Words:
We have tried our best to provide you with all the possible solutions to fix the above error. You can also get help for Errors and difficulties in your Minecraft game like Majong screen freeze, Not responding error or Minecraft Shaders installation issues. Because after in-depth research of the above issue, our team members currently can handle any problem with Minecraft.
So, Feel free to ask any questions and share your valuable thoughts with us in the comments section below. We’ll be glad to hear from you. An Existing Connection Was Forcibly Closed by the Remote Host An Existing Connection Was Forcibly Closed by the Remote Host An Existing Connection Was Forcibly Closed by the Remote Host An Existing Connection Was Forcibly Closed by the Remote Host An Existing Connection Was Forcibly Closed by the Remote Host
Время на прочтение
14 мин
Количество просмотров 2.4K
Нормальные герои всегда идут в обход
Айболит 66
Думаю что многие встречались с необъяснимым поведением компонентов 1С, подсистем ОС, драйверов, СУБД которое видно в трассировке, но трассировка не отвечала на главный вопрос почему? Т.е. по документации должно работать, но не работает.
Несмотря на все более возрастающее движение за системы с открытым кодом, всегда есть что-то к чему нет исходников, либо нет компетенций, ресурсов в них разбираться. Ну даже если Вы найдете причину в открытом коде – скажем PostgreSQL, чтобы это исправить нужны хорошие навыки и компетенции. И все дороги получается ведут по пути Workaround (обходной путь), поскольку решение службы поддержки ждать дольше.
Более тяжелым случаем являются ограничения платформы (напр ограничения 1С при горизонтальном маштабировании см Язык мой враг мой ) , которые тоже приходится обходить.
В английском закрепился термин и ему посвящена статься в Wiki , где его связывают с анти-паттерном
Workaround wiki
Надо сказать Workaround не обязательно костыль, или заплата, но он всегда несет в себе какие-то минусы – ведь мы действуем обходным путем
Термины не возникают на пустом месте – значит сложилась предыстория, приемы, методы которые нужно было как то назвать. Например названия трюков на вейкборде Ollie, Wake to wake и т.д… это ниточка за которой тянется техника, подводящие упражнения и многое другое
Умение создавать workaround жизненно важно для систем,
-
У которых маленькие технологические окна . Например наша, где роботы работают почти круглосуточно 1с БодиПозитив и с высокой нагрузкой.
-
Устаревшие системы, которые сняты с поддержки
-
Системы с высокой нагрузкой, использующие горизонтальное маштабирование, где проявляются эффекты бутылочных горлышек .
-
Системы крупных вендоров, где не будет мгновенной реакции службы поддержки (Конечно Вы можете попробовать расширенную поддержку 1С Корп, но руководство будет смотреть на Вас, а не на поддержку 1С 😉 )
-
Я надеюсь в комментариях добавят еще интересные кейсы
Разберем методы создания Workaround на реальных историях. Предлагаемая классификация методов наверняка не полная и в комментариях к статье добавят много нового
История №1 Как MS SQL случайным образом отключал соединения 1С (актуально и сейчас)
Все началось с жалоб пользователей на ошибки сверки с Backoffice при закрытии дня. Анализ показал, что некоторые фоновые задания, применяющиеся при расчетах с горизонтальным маштабированием завершались некорректно. Надо заметить что 1С сейчас хранит историю только по 1000 последних фоновых заданий, и мы создали расширенное логгирование в конфигурации для отслеживания таких ситуаций. Подробнее о проблеме можно посмотреть тут Язык мой Враг мой .
В 1С проблему можно локализовать анализом тех журнала, а там заметили следующее
16:42.3540-0,SCOM,2,process=rphost,t:clientID=2352,Func='setSrcProcessName(MyDB, MyDB)'
16:42.4481-0,EXCP,2,process=rphost,p:processName= MyDB,t:clientID=2352,t:applicationName=BackgroundJob,t:connectID=14847,Exception=DataBaseException,Descr='Ошибка СУБД:
Microsoft SQL Server Native Client 11.0: TCP Provider: An existing connection was forcibly closed by the remote host.
HRESULT=80004005, HRESULT=80004005, SQLSrvr: SQLSTATE=08001, state=1, Severity=10, native=10054, line=0
Удивительно – но MS SQL сам отключает коннекты «случайным образом»? Анализ логов MS SQL Операционной системы ничего не дал, в какие то критические режимы MS SQL не входил.
Выхода было два либо копать глубже с трассировкой на уровне стека TCP и MS SQL Profiler либо задать вопрос Google – возможно он не даст прямое решение, но какую то идею
К счастью Google сразу вывел на статью Microsoft
TLS Connection forcibly closed где была описана ошибка в TLS протоколах и Workaround для нашей версии Windows – отключить TLS_DHE_* в групповых политиках.
В данном случае workaround сделан по принципу
Не работает – измени окружение
Поскольку проблемные методы шифрования были убраны из политик и оставлены работающие – окружение 1С – MS SQL сервер изменилось
У кого-то возникнет вопрос – а как действовать если Google не вывел на прямой ответ?
Многие бросаются ставить все возможные патчи — ковровой бомбардировкой на ОС, SQL Server , 1С. Мне не нравится этот метод поскольку они могут привести к другим проблемам, если длительно не выдержаны и протестированы на резервном контуреконтуре тестирования. Кроме того, в рассматриваемом случае патчи есть только для новых ОС
В рассматриваемом случае можно еще больше локализовать проблему – ведь ошибка TCP провайдера не означает что проблема на транспортном уровне модели OSI . Нужно проверять еще прикладной , представления , сеансовый. В документации по ошибке есть пример network traсe который позволяет ее выявить и судя по всему это сделано WireShark Habr o WireShark и придется привлечь системных адмнистраторов.
Если повезет можно быстро понять что дело на уровне шифрования TLS , но если нет возможности заниматься анализом, можно поступить по другому.
В нашем случае ошибка регистрируется периодически для фоновых заданий при взаимодействии сервера приложений 1С и SQL сервера и вредит она фоновым заданиям
Вариант 1. А что если сделать повторный запуск фоновых заданий в 1С, если оно не доработало? В целом это нормальный вариант, который повысит выживаемость 1С в нестабильной среде при любых таких плавающих проблемах. Такой подход называется
Не работает — Измени подсистему и ее поведение
Вариант 2 Перенастроить кластер 1С на сервер с MS SQL и включить Shared memory protocol . Радикальная смена стека протоколов поможет обойти проблему по пути «Не работает – измени окружение». Да на нагрузка на оборудование возрастет, но зато прикладные проблемы уйдут
Сделав обходной путь можно спокойно докапываться до сути проблемы на резервном кластере.
Для крупных систем наличие резервного дублирующего контура жизненно необходимо чтобы
-
тестировать все патчи и обновления
-
воспроизводить, исследовать, взаимодействовать со службой поддержки по таким проблемам
-
подготовить среду, где эти проблемы не возникают (напр новая операционная система, новый набор патчей и т.д.)
История №2 Как ускорить запись в регистр сведений 1С? Почти Антипаттерн пока 1С не исправит
При использовании горизонтального маштабирования на уровне кластера 1С можно создать множество параллельных задач, которые постят движения в регистры, импортируют операции, и можно даже параллельно считать статьи баланса. Однако узким местом в 1С является запись в регистры (подробнее изложено в статье Язык мой Враг мой).
Допустим нам нужно импортировать операций по которым 6 миллионов изменений за день, а уникальных операций около миллиона в день. Изменения поступают по шине RabbitMQ.
Построим регистры СУУ_АгрегированнаяСделкаКП (Индексы по измерениям , поле Удалено, Дата)
СУУ_НеобязательныеРеквизитыДляАгрегированныхОпераций (Индексы по измерениям)
С импортом новых операций проблем не возникнет, их можно записывать в регистры сведений сразу набором по 1000 шт за один раз с распараллеливанием.
Но если мы захотим обновить 1000 операций , то это можно сделать только по отдельным ИдИсхСистемы — поскольку фильтр отбора по измерений 1С работает только на равенство везде т.е. получим 1000 отдельных вызовов .Записать()
Метаданные типа Документ еще хуже – документы можно записывать по одному , а не несколько в крупном DML.
Даже если эти записи распараллелены на 40 потоков – SQL сервер будет под атакой тысяч небольших DML операций со всеми вытекающимидля производительности.
Добавлю, что при обновлении нужно решать проблему правильной записи версий, чтобы версия 1 не записалась поверх версии 2
Как укрупнить DML в таких условиях если хочется остаться в рамках 1С?
Изменить свое отношение к проблеме, решай другую проблему
Обновление большого количества операций имеющих изменения, это затратная по производительности задача даже если использовать конструкции типа Merge в SQL, ведь приходится обновлять не только таблицы, но и индексы.
Но ведь можно ничего не обновлять, а писать сразу версии! Сделаем регистры сведений периодическими и будем писать версии (версия в стандартном поле Период )! Главное чтобы версия была одинакова и у реквизитов и операций . Поле Период по факту будет временем начала записи пакета из 1000 операцийнеобязательных реквизитов
Да усложнится отбор операций для последующей обработки — ведь нам нужно отбирать последние версии операций и соединять их при необходимости с последними версиями необязательных реквизитов. Но зато мы можем организовать импорт максимально быстро в данном окружении
Анонс: Эффективное написание запросов в этом случае тема отдельной статьи
1С и в этом случае добавляет барьеров в виде неэффективных соединений с временными таблицами ( подробно тут 1С Миссия невыполнима. Общие реквизиты разделители против временных таблиц ) но в целом задача решается, просто изменением подхода к проблеме.
Хочется еще быстрее – тогда пишите в регистр сведений напрямую минуя 1С , но это уже другая история.
История №3 Как рабочий процесс 1С терял контекст драйвера Oracle
Дело было в 2014 году , мы активно распараллеливали импорта фоновыми заданиями задачи импорта. Появилась задача импортировать операции с базы на Oracle в 1С, обращение к серверу Oracle шло с использованием драйвера Oracle12c, на сервере приложений. В процессе эксплуатации начали регистрироваться сбои. Текст ошибки не помню, но суть была в потере контекста для определения параметров Oracle_home, причем периодически, а не постоянно.
Замечу, для драйверов Microsoft для Oracle ничего подобного не наблюдалось, но по разным причинам нужно было использовать родной драйвер Oracle
Тут сложно было сказать, кто виноват 1С или Oracle , но было ясно, что более глубокая трассировка вряд ли приведет к решению, а общение со службой поддержки затянется. Поэтому применили принцип
Не работает — Измени подсистему и ее поведение
Подсистема работы с внешними источниками в 1С тогда еще была с ограниченным функционалом, поэтому использовали ADODB
Сначала была попытка соединится правильно, но если не удавалось создавали Ole вызов клиента 1С на сервере из фонового задания, и уже через Ole брали данные. Обходной вариант выглядел так (код сократил для удобочитаемости)
ADOConnection = СУУ_СоздатьОбъектАДО(ИДВызова, "ADODB.Connection");
Если ADOConnection = Неопределено Тогда
Возврат Неопределено;
КонецЕсли;
ОбъектОЛЕ = Неопределено;
СоединениеУстановлено = Ложь;
// установка соединения
Попытка
ADOConnection.Open(СтрокаСоединения);
СоединениеУстановлено = Истина;
Исключение
// Ошибка
КонецПопытки;
Если НЕ СоединениеУстановлено Тогда
// сюда дойдем, если при подключении через родной объект ADODB.Connection произошла ошибка
// на ORACLE x64 + 1С x64 + Windows x64 есть ошибка - после какого-то количества успешных подключений возникает ошибка.
//решение проблемы не найден. есть только обход:
//если соединение не установилось из конкретно этой базы в серверном режиме, то нужно получить соединение
//из клиента 1С, который всегда x32
//для этого написана микро-конфа с тремя экспортными методами:
//ADOConnectionObj();
//ADOCommandObj();
//ADORecordsetObj();
//фишка - если получили Connection таким образом, то и Command нужно тоже получить из 32-х разрядного клиента,
//иначе будет ошибка на этом моменте: Command.ActiveConnection = ADOConnection;
ОбъектОЛЕ = Неопределено;
Попытка
ОбъектОЛЕ = Новый COMобъект("V82.Application");
ОбъектОЛЕ.Visible=Ложь;
ОбъектОЛЕ.Connect("File=""C:1CComSrv""; Usr="""";Pwd="""";");
ОбъектОЛЕ.Visible=Ложь;
СоединениеОЛЕ = Истина;
Исключение
//Сообщаем об ошибке
УничтожитьОбъектАДО(ADOConnection);
УничтожитьОбъектОЛЕ(ОбъектОЛЕ);
Возврат Неопределено;
КонецПопытки;
Некрасиво получается, а что делать?
Не работает – убери подсистему из окружения
Более красивый вариант – создать DBLink с Oracle на MSSQL сервере, где находилась база 1С , и обращаться уже к DBlink используя только драйвера Microsoft, так в принципе можно сделать более универсальным код работы с внешними СУБД. Минус – слишком много слоев и сложный маршрут по сети (1С сервер – MS Sql сервер – Oracle сервер).
История №4 из Java
Я не эксперт в Java, но писал небольшие приложения для склеивания 1С с различными шинами, приложениями, для администрирования СУБД и т.д. Ведь когда что-то в 1С невозможно проще найти это в мире Java и интегрировать.
При попытке написать приложение с использованием JSF + Glassfish обнаружил что связка категорически не хочет работать даже с Hello word см тут
Мой наивный вопрос на StackOverflow
Я не смог найти рецептов понять причину проблемы, и пришлось решить сменой сервера приложений Glassfish на Payara. Да вот так радикально. Вообще качество решений на Java это тема отдельной статьи. Количество конкурирующих решений размывает качество тестирования на конечных потребителях. И после этого начинаешь очень ценить порядок и предсказуемость в платформе 1С 😊.
Очень интересно будет увидеть в комментариях случаи и решения не укладывающиеся в данную классификацию и другие методы создания Workaround. Присоединяйтесь к нашему телеграмм каналу t.me/Chat1CUnlimited.